Java Swing Program to demonstrate JLabel with Icon
Program
import javax.swing.*;
public class JLabelIconDemo
{
public static void main(String args[])
{
JFrame frm = new JFrame("JLabel Demo");
JLabel lblUserName, lblPassword;
ImageIcon icoUserName, icoPassword;
icoUserName = new ImageIcon("username.png");
lblUserName = new JLabel(icoUserName);
lblUserName.setBounds(0, 0, 100, 50);
icoPassword = new ImageIcon("password.png");
lblPassword = new JLabel(icoPassword);
lblPassword.setBounds(0, 50, 100, 50);
frm.add(lblUserName);
frm.add(lblPassword);
frm.setSize(300, 300);
frm.setLayout(null);
frm.setVisible(true);
frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
This Java Swing program demonstrates how to use JLabel
with icons (ImageIcon
). It displays two labels, each with an image icon representing a "username" and "password" label.
-
Import Swing Package:
- The
javax.swing.*
package is imported to useJFrame
,JLabel
, andImageIcon
components.
- The
-
Main Components:
JFrame
: A container used to create the main application window.JLabel
: A component used to display text or images. In this case, it is used to display images (icons).ImageIcon
: A class used to create an icon from an image file, which can be displayed on a label.
-
Creating a JFrame:
- A
JFrame
object (frm
) is created with the title "JLabel Demo". - The
setSize(300, 300)
method defines the window size (300x300 pixels). setLayout(null)
allows for absolute positioning of components within the frame.
- A
-
Creating Image Icons:
ImageIcon icoUserName = new ImageIcon("username.png")
: This creates anImageIcon
from an image file (e.g.,username.png
).ImageIcon icoPassword = new ImageIcon("password.png")
: This creates anotherImageIcon
from the image filepassword.png
.
-
Creating JLabels with Icons:
lblUserName = new JLabel(icoUserName)
: This creates aJLabel
that will display theicoUserName
image.lblPassword = new JLabel(icoPassword)
: This creates anotherJLabel
that will display theicoPassword
image.- The
setBounds(x, y, width, height)
method is used to position and size the labels on the frame.
-
Adding Labels to JFrame:
- Both
JLabel
components (lblUserName
andlblPassword
) are added to theJFrame
usingadd()
method.
- Both
-
Frame Visibility and Behavior:
- The
setVisible(true)
method makes the frame visible on the screen. - The
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
method ensures the program terminates when the window is closed.
- The
-
Key Swing Methods Used:
JLabel(ImageIcon icon)
: Creates a label with an icon (image).setBounds(int x, int y, int width, int height)
: Positions and sizes the label in the frame.add(Component comp)
: Adds components like labels to the frame.setDefaultCloseOperation(int operation)
: Defines the window's close behavior (e.g., exit the program when closed).setVisible(boolean visibility)
: Makes the frame visible.
Output
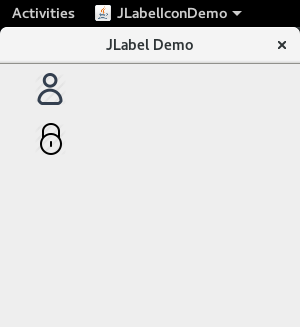