Java Swing Program to demonstrate JLabel with Text and Icon
Program
import javax.swing.*;
public class JLabelTextIconDemo
{
public static void main(String args[])
{
JFrame frm = new JFrame("JLabel Demo");
JLabel lblUserName, lblPassword;
ImageIcon icoUserName, icoPassword;
icoUserName = new ImageIcon("username.png");
lblUserName = new JLabel();
lblUserName.setText("User Name");
lblUserName.setIcon(icoUserName);
lblUserName.setBounds(0, 0, 150, 50);
icoPassword = new ImageIcon("password.png");
lblPassword = new JLabel();
lblPassword.setText("Password");
lblPassword.setIcon(icoPassword);
lblPassword.setBounds(0, 50, 150, 50);
frm.add(lblUserName);
frm.add(lblPassword);
frm.setSize(300, 300);
frm.setLayout(null);
frm.setVisible(true);
frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
This Java Swing program demonstrates how to use a JLabel
with both text and an icon in a graphical user interface (GUI). It displays two labels, each containing a combination of text ("User Name" and "Password") and an associated image icon.
-
Imports:
- The
javax.swing.*
package is imported to utilize theJFrame
,JLabel
, andImageIcon
classes.
- The
-
Creating the JFrame:
JFrame frm = new JFrame("JLabel Demo")
: This creates a new window titled "JLabel Demo" using theJFrame
class.setSize(300, 300)
: This method sets the size of the frame to 300 pixels by 300 pixels.setLayout(null)
: Thenull
layout manager allows you to manually set the positions of components in the frame.setVisible(true)
: Makes the frame visible on the screen.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
: Ensures that the program terminates when the window is closed.
-
Creating Image Icons:
ImageIcon icoUserName = new ImageIcon("username.png")
: This loads an image (e.g., "username.png") as an icon.ImageIcon icoPassword = new ImageIcon("password.png")
: This loads another image (e.g., "password.png") for the second label.
-
Creating JLabel with Text and Icon:
lblUserName.setText("User Name")
: This sets the text "User Name" for the first label.lblUserName.setIcon(icoUserName)
: This sets the image icon (loaded from"username.png"
) to be displayed beside the text on the label.lblPassword.setText("Password")
: This sets the text "Password" for the second label.lblPassword.setIcon(icoPassword)
: This sets the image icon (loaded from"password.png"
) for the second label.
-
Positioning the Labels:
setBounds(x, y, width, height)
: This method positions and sizes the labels within the frame.- The
lblUserName
label is placed at (0, 0) with width 150 and height 50. - The
lblPassword
label is placed at (0, 50) with width 150 and height 50.
- The
-
Adding Labels to JFrame:
- Both
lblUserName
andlblPassword
are added to the frame usingadd()
.
- Both
-
Key Swing Methods Used:
JLabel()
: Creates an empty label.setText(String text)
: Sets the text for the label.setIcon(Icon icon)
: Sets an image icon for the label.setBounds(int x, int y, int width, int height)
: Positions and sizes the label.add(Component component)
: Adds the label to the JFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
: Specifies that the program should exit when the window is closed.
Output
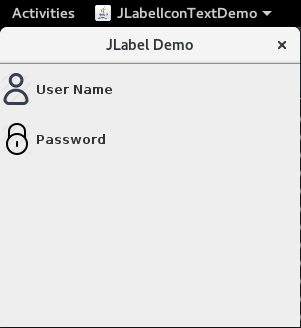