Java Swing Program to demonstrate Jlabel
Program
import javax.swing.*;
public class JLabelDemo
{
public static void main(String args[])
{
JFrame frm = new JFrame("JLabel Demo");
JLabel lblUserName, lblPassword;
lblUserName = new JLabel("User Name:");
lblUserName.setBounds(0, 0, 100, 50);
lblPassword = new JLabel("Password:");
lblPassword.setBounds(0, 50, 100, 50);
frm.add(lblUserName);
frm.add(lblPassword);
frm.setSize(300, 300);
frm.setLayout(null);
frm.setVisible(true);
frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
This Java Swing program demonstrates the use of JLabel
, a component in the Swing library used for displaying text or images.
-
Import Swing Package:
- The
javax.swing.*
package is imported to use Swing components likeJFrame
andJLabel
.
- The
-
Main Components:
JFrame
: A container that acts as the main window for the application.JLabel
: A Swing component used to display static text or images.
-
Creating a JFrame:
- A
JFrame
object (frm
) is created with the title "JLabel Demo". - The
setSize()
method is used to set the frame dimensions (300x300 pixels). - The
setLayout(null)
method allows absolute positioning of components.
- A
-
Creating JLabels:
- Two
JLabel
objects (lblUserName
andlblPassword
) are created with text "User Name:" and "Password:", respectively. - The
setBounds()
method sets the position and dimensions for each label. For example:lblUserName
starts at (0, 0) with width 100 and height 50 pixels.lblPassword
starts at (0, 50) with width 100 and height 50 pixels.
- Two
-
Adding Labels to JFrame:
- Both
JLabel
objects are added to theJFrame
using theadd()
method.
- Both
-
Frame Visibility and Behavior:
- The
setVisible(true)
method makes the frame visible. - The
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
ensures the application exits when the window is closed.
- The
-
Key Swing Methods Used:
JLabel(String text)
: Creates a label with the specified text.setBounds(int x, int y, int width, int height)
: Sets the position and size of the label.add(Component comp)
: Adds a component (e.g., JLabel) to the frame.setLayout(LayoutManager mgr)
: Specifies the layout manager;null
enables absolute positioning.setDefaultCloseOperation(int operation)
: Defines the behavior when the window is closed.
Output
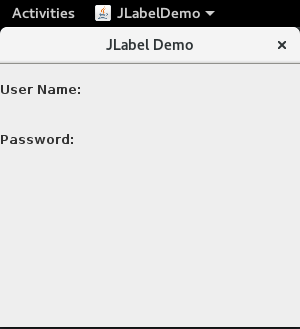