Java Swing Program to demonstrate JButton with Text and Icon
Program
import javax.swing.*;
public class JButtonTextIconDemo
{
public static void main(String[] args)
{
JFrame frm = new JFrame("JButton Demo");
JButton btnSubmit;
ImageIcon icoSubmit;
icoSubmit = new ImageIcon("login.png");
btnSubmit = new JButton();
btnSubmit.setText("Login");
btnSubmit.setIcon(icoSubmit);
btnSubmit.setBounds(0, 0, 100, 50);
frm.add(btnSubmit);
frm.setSize(300, 300);
frm.setLayout(null);
frm.setVisible(true);
frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
This Java Swing program demonstrates how to create a JButton
that combines both text and an icon. The button will display a label with the text "Login" alongside an image icon (in this case, "login.png"). This type of button is useful for providing both visual and textual cues to the user in a graphical user interface.
-
Imports:
- The program imports the
javax.swing.*
package to access the Swing components likeJFrame
,JButton
, andImageIcon
.
- The program imports the
-
Creating the JFrame:
JFrame frm = new JFrame("JButton Demo")
: Creates a window (frame) with the title "JButton Demo".frm.setSize(300, 300)
: Sets the window size to 300x300 pixels.frm.setLayout(null)
: Disables the default layout manager, allowing manual positioning of the components within the frame.frm.setVisible(true)
: Makes the window visible when the program is executed.frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
: Ensures that the program terminates when the window is closed.
-
Creating the JButton with Text and Icon:
ImageIcon icoSubmit = new ImageIcon("login.png");
: Creates anImageIcon
from the file "login.png". This image should exist in the directory where the program is running.btnSubmit = new JButton();
: Creates an emptyJButton
object.btnSubmit.setText("Login");
: Sets the text of the button to "Login".btnSubmit.setIcon(icoSubmit);
: Sets the icon of the button to the previously createdImageIcon
(the image "login.png").btnSubmit.setBounds(0, 0, 100, 50);
: Sets the position and size of the button within the frame. The button is placed at coordinates(0, 0)
and has a width of 100 pixels and a height of 50 pixels.
-
Adding the JButton to the JFrame:
frm.add(btnSubmit);
: Adds the button to the frame so that it will be displayed when the program runs.
-
Key Swing Methods Used:
ImageIcon("imagePath")
: Creates anImageIcon
from the specified file path (in this case, "login.png"). The image file must be located in the program's working directory or the specified path.JButton()
: Creates an empty button that can later be customized with text and icons.setText(String text)
: Sets the text that appears on the button. In this case, it will display "Login".setIcon(Icon icon)
: Sets the icon (image) displayed on the button. The image used is from the "login.png" file.setBounds(x, y, width, height)
: Specifies the position and size of the button in the window. It is placed at(0, 0)
and has dimensions of 100x50 pixels.add(Component component)
: Adds the button to the JFrame so that it can be displayed in the graphical user interface.
Output
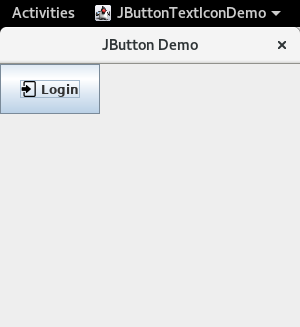