Java Swing Program to demonstrate JButton with Icon
Program
import javax.swing.*;
public class JButtonIconDemo
{
public static void main(String args[])
{
JFrame frm = new JFrame("JButton Demo");
JButton btnSubmit;
ImageIcon icoSubmit;
icoSubmit = new ImageIcon("login.png");
btnSubmit = new JButton(icoSubmit);
btnSubmit.setBounds(0, 0, 100, 50);
frm.add(btnSubmit);
frm.setSize(300, 300);
frm.setLayout(null);
frm.setVisible(true);
frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
This Java Swing program demonstrates how to create a JButton
that displays an icon (an image) rather than text. It creates a window (JFrame) with a JButton
that contains an image, which can be used as an interactive button in the GUI.
-
Imports:
- The program imports the
javax.swing.*
package to use Swing components likeJFrame
,JButton
, andImageIcon
.
- The program imports the
-
Creating the JFrame:
JFrame frm = new JFrame("JButton Demo")
: Creates a frame (window) with the title "JButton Demo".frm.setSize(300, 300)
: Sets the size of the window to 300x300 pixels.frm.setLayout(null)
: Disables the default layout manager, allowing manual positioning of the button.frm.setVisible(true)
: Makes the window visible when the program runs.frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
: Ensures that the program will terminate when the window is closed.
-
Creating the JButton with an Icon:
ImageIcon icoSubmit = new ImageIcon("login.png");
: Creates anImageIcon
from the file "login.png". This image file should exist in the directory where the program is running.btnSubmit = new JButton(icoSubmit);
: Creates aJButton
and sets its icon to the previously createdImageIcon
. The text of the button is omitted, and only the image is displayed on the button.btnSubmit.setBounds(0, 0, 100, 50);
: Sets the position and size of the button within the frame. The button is placed at the coordinates(0, 0)
on the frame and has a width of 100 pixels and height of 50 pixels.
-
Adding the JButton to JFrame:
frm.add(btnSubmit);
: Adds the button to the frame so that it will appear in the GUI.
-
Key Swing Methods Used:
ImageIcon("imagePath")
: This creates an image icon from the specified image file path (e.g., "login.png"). Ensure the image is in the correct directory for the program to locate it.JButton(Icon icon)
: This creates aJButton
with the specified icon. In this case, instead of setting the text of the button, the image is displayed.setBounds(x, y, width, height)
: This method sets the position and size of the button on the frame. The button is placed at(0, 0)
and has a width of 100 pixels and a height of 50 pixels.add(Component component)
: Adds the button to the frame so that it is displayed in the GUI.
Output
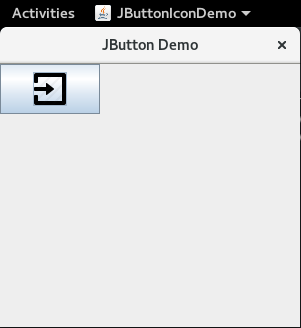