Java Swing Program to demonstrate JButton Action TextField
Program
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JTextField;
import javax.swing.*;
import java.awt.event.*;
public class JButtonActionTextBox{
public static void main(String[] args) {
JFrame frm = new JFrame("JButton Action Listener");
JPanel pnl = new JPanel();
JTextField txtDisplay = new JTextField(20);
txtDisplay.setBounds(50, 50, 150, 20);
JButton btnSubmit = new JButton("Click Here");
btnSubmit.setBounds(50, 200, 100, 20);
btnSubmit.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
txtDisplay.setText("Welcome to www.oodlescoop.com");
}
});
pnl.add(txtDisplay);
pnl.add(btnSubmit);
frm.add(pnl);
frm.setSize(300, 300);
frm.setVisible(true);
frm.setLayout(null);
frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
This Java Swing program demonstrates the use of a JButton with an ActionListener to perform an action on a JTextField. Here's a brief explanation:
-
JFrame Setup:
- A
JFrame
namedfrm
is created with the title "JButton Action Listener." - The
JFrame
is set to close the application when the window is closed usingsetDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
.
- A
-
JPanel:
- A
JPanel
namedpnl
is added to the frame to hold the components.
- A
-
JTextField:
- A
JTextField
namedtxtDisplay
is created with a width of 20 columns for text input/display. - Its bounds are set using
setBounds
, though this is unnecessary since the program usesJPanel
(which uses a default layout manager).
- A
-
JButton:
- A
JButton
namedbtnSubmit
is created with the label "Click Here." - An
ActionListener
is added to the button usingaddActionListener
. When the button is clicked, thetxtDisplay
text is updated to display the message "Welcome to www.oodlescoop.com".
- A
-
Adding Components:
- Both the
txtDisplay
andbtnSubmit
components are added to theJPanel
. - The
JPanel
is then added to theJFrame
.
- Both the
-
Display Settings:
- The
JFrame
size is set to300x300
. - The
JFrame
is made visible withsetVisible(true)
.
- The
Output
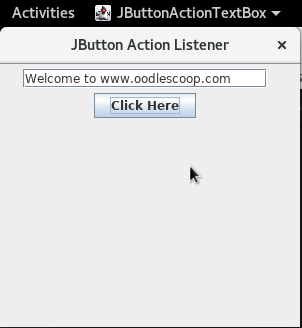