Java Swing Program to demonstrate JTextField
Program
import javax.swing.*;
public class JTextFieldDemo
{
public static void main(String args[])
{
JFrame frm = new JFrame("JTextField Demo");
JTextField txtUserName, txtPassword;
txtUserName = new JTextField();
txtUserName.setBounds(0, 0, 100, 20);
txtPassword = new JTextField();
txtPassword.setBounds(0, 20, 100, 20);
frm.add(txtUserName);
frm.add(txtPassword);
frm.setSize(300, 300);
frm.setLayout(null);
frm.setVisible(true);
frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
This Java Swing program demonstrates how to create and use JTextField
components in a GUI (Graphical User Interface). The JTextField
allows the user to input text, and in this program, two text fields are created — one for the username and one for the password.
-
Imports:
- The program imports
javax.swing.*
, which is necessary to use Swing components likeJFrame
andJTextField
.
- The program imports
-
Creating the JFrame:
JFrame frm = new JFrame("JTextField Demo")
: This creates a window (frame) titled "JTextField Demo".frm.setSize(300, 300)
: Sets the size of the frame to 300x300 pixels.frm.setLayout(null)
: This disables any layout manager and allows manual positioning of components inside the frame.frm.setVisible(true)
: Makes the frame visible when the program is run.frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
: Ensures that the program terminates when the window is closed.
-
Creating JTextField Components:
txtUserName = new JTextField()
: Creates a text field where the user can type the username.txtPassword = new JTextField()
: Creates a text field where the user can type the password.
-
Positioning the JTextFields:
txtUserName.setBounds(0, 0, 100, 20)
: Sets the position and size of thetxtUserName
field. It will appear at the coordinates (0, 0) with a width of 100 pixels and a height of 20 pixels.txtPassword.setBounds(0, 20, 100, 20)
: Sets the position and size of thetxtPassword
field. It will appear just below the username field at coordinates (0, 20).
-
Adding Components to the JFrame:
frm.add(txtUserName)
andfrm.add(txtPassword)
: These lines add the two text fields to the frame.
-
Key Swing Methods Used:
JTextField()
: Creates an empty text field for user input.setBounds(x, y, width, height)
: Defines the position and size of the text field.add(Component component)
: Adds the text fields to the JFrame.setVisible(true)
: Makes the frame visible on the screen.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
: Ensures that the program will exit when the window is closed.
Output
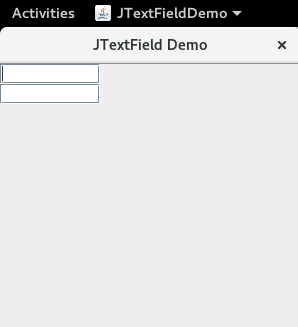