Java Swing Program to demonstrate JCheckBox with Text
Program
import javax.swing.*;
public class JCheckBoxDemo
{
public static void main(String[] args)
{
JFrame frm = new JFrame("JCheckBox Demo");
JCheckBox chkbxMilk = new JCheckBox();
chkbxMilk.setText("Milk");
chkbxMilk.setSelected(true);
chkbxMilk.setBounds(0, 0, 100, 50);
JCheckBox chkbxCoffee = new JCheckBox();
chkbxCoffee.setText("Coffee");
chkbxCoffee.setBounds(0, 50, 100, 50);
JCheckBox chkbxTea = new JCheckBox();
chkbxTea.setText("Tea");
chkbxTea.setBounds(0, 100, 100, 50);
frm.add(chkbxMilk);
frm.add(chkbxCoffee);
frm.add(chkbxTea);
frm.setSize(300, 300);
frm.setLayout(null);
frm.setVisible(true);
frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
This Java Swing program demonstrates the use of JCheckBox
components to create a simple UI with selectable options. The program creates a simple GUI with three checkboxes allowing users to select or deselect options.
-
JFrame Setup:
- A
JFrame
namedfrm
is created with the title "JCheckBox Demo." - The
JFrame
is set to close the application when the window is closed usingsetDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
.
- A
-
JCheckBox Components:
- Three
JCheckBox
components are created to represent options ("Milk," "Coffee," and "Tea"). - Each checkbox:
- Is created using
new JCheckBox()
. - Has its label set using
setText()
. - Has a specific position and size set using
setBounds()
.
- Is created using
- The checkbox for "Milk" is pre-selected using
setSelected(true)
.
- Three
-
Adding Checkboxes to JFrame:
- Each
JCheckBox
is added to the frame usingfrm.add()
. - The application uses a default static behavior with no event handling for the checkboxes. To add interactivity, event listeners (e.g.,
ItemListener
) can be used.
- Each
-
Frame Configuration:
- The frame size is set to
300x300
pixels usingsetSize()
. - A
null
layout is used for manual positioning of components withsetLayout(null)
. - The frame is made visible with
setVisible(true)
. - Manual positioning is achieved using
setBounds()
, though using layout managers (likeFlowLayout
orBoxLayout
) would be a better approach for dynamic UIs.
- The frame size is set to
Output
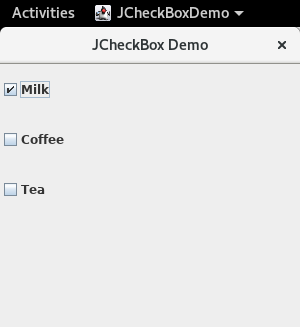