Features of Java
Java is one of the most popular and widely-used programming languages, known for its powerful features that make development efficient, secure, and platform-independent. Here’s a breakdown of the core features that contribute to Java’s success:
1. Simple
Java was designed to be easy to learn and use. Its syntax is clean and readable, inspired by C/C++ but simplified to eliminate complex and error-prone features like pointers and multiple inheritance. This makes Java accessible for beginners and efficient for experienced developers.
2. Object-Oriented
Java is a fully object-oriented programming (OOP) language. Everything in Java is associated with classes and objects, which makes the code modular, reusable, and easier to maintain. Core OOP concepts in Java include inheritance, encapsulation, abstraction, and polymorphism.
3. Portable
Java programs are portable across platforms thanks to the Java compiler generating bytecode, which can run on any device equipped with the Java Virtual Machine (JVM). This eliminates dependency on underlying hardware or operating systems.
4. Platform Independent
Java achieves platform independence through the use of JVM. Once a Java program is compiled into bytecode, it can be executed on any system that has a compatible JVM, regardless of the operating system.
"Write Once, Run Anywhere (WORA)" is Java’s key mantra, which highlights its platform-independent nature.
5. Secure
Security is a primary concern in Java. It provides several built-in features such as bytecode verification, runtime security checks, and a robust security API. Java applications run in a controlled environment (sandbox), which restricts unauthorized access to system resources.
6. Robust
Java emphasizes early error checking, runtime checking, and memory management to ensure code reliability. Features like automatic garbage collection, exception handling, and strong type checking contribute to Java's robustness.
7. Architecture Neutral
Java code does not rely on underlying hardware architecture. The compiled bytecode is independent of the architecture of the host system, allowing Java programs to be executed on any machine with a JVM, without requiring recompilation.
8. Dynamic
Java supports dynamic linking of new classes, methods, and objects. At runtime, classes are loaded into memory as needed, and Java can even query class structures using reflection. This enables the creation of highly flexible and extensible applications.
9. Compiled and Interpreted
Java combines both compilation and interpretation. First, the Java compiler (javac
) compiles the source code into bytecode. Then, the JVM interprets or compiles (via Just-In-Time compilation) the bytecode into machine code for execution, providing both flexibility and speed.
10. High Performance
Although interpreted, Java achieves high performance through:
- Just-In-Time (JIT) Compilation – Converts frequently used bytecode into native machine code at runtime.
- Efficient memory management – Through garbage collection and runtime optimizations.
Java is not as fast as C/C++, but its performance is sufficient for most applications, and improvements in JVM technology continue to narrow the gap.
11. Multi-threaded
Java provides built-in support for multi-threading, which allows the execution of multiple parts of a program simultaneously. This is especially useful for developing interactive, high-performance applications like games, animations, and server-based systems.
12. Distributed
Java makes it easy to create applications that can run on networks. With built-in libraries like Remote Method Invocation (RMI) and support for network protocols (e.g., HTTP, FTP, TCP/IP), Java facilitates the development of distributed systems and cloud-based applications.
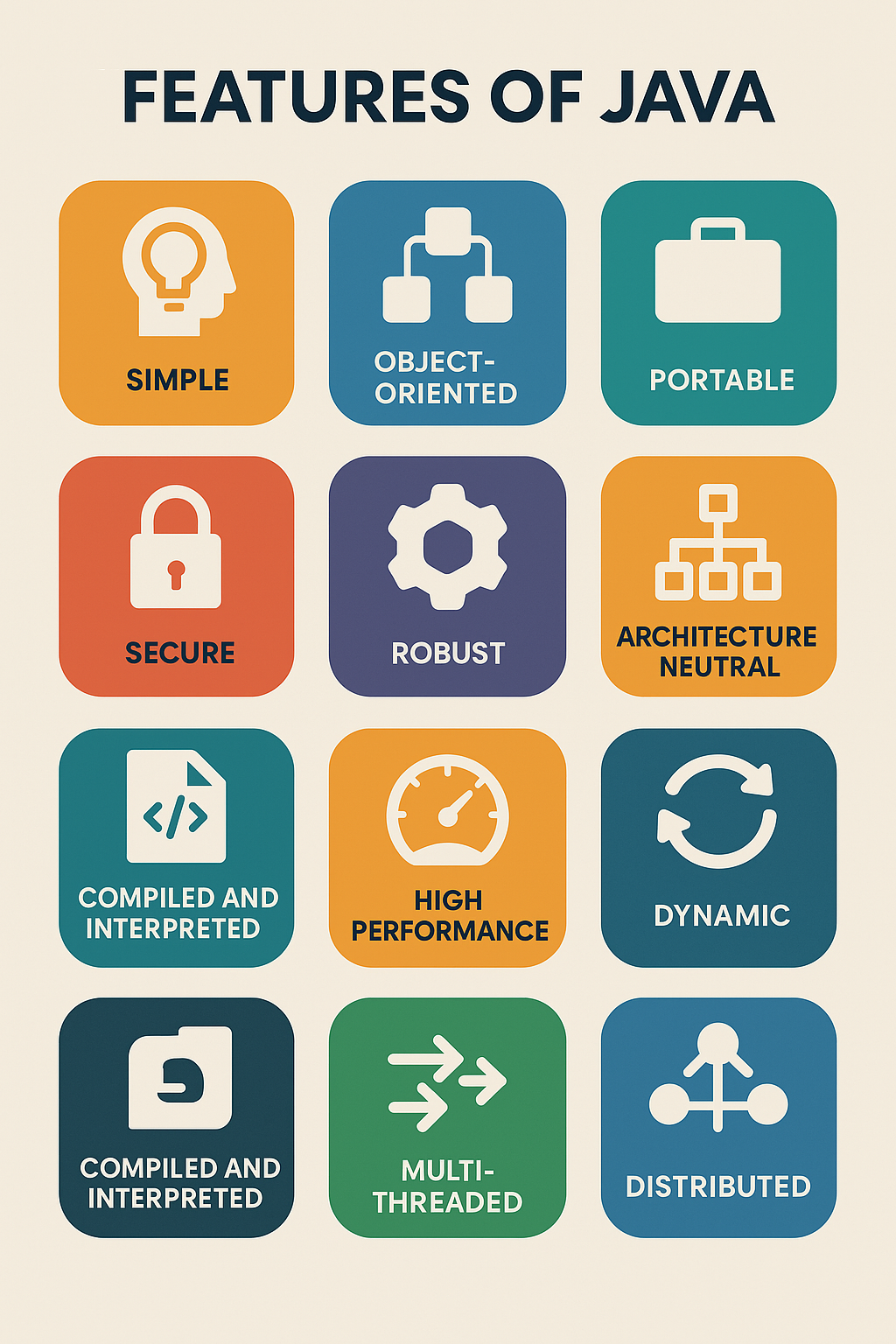